Tokenization is an important step in NLP data preprocessing that involves breaking the text into smaller units called tokens. These tokens can be individual words, characters, or subwords depending on the tokenization strategy. This tutorial provides an in-depth exploration of tokenization techniques, their importance in NLP tasks, and practical examples using Python and popular NLP libraries.
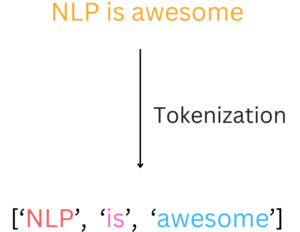
Why tokenization/need of tokenization
Tokenization plays a crucial role in natural language processing (NLP) for several reasons:
- Text Segmentation: Tokenization splits the text into smaller units that facilitate in further preprocessing and analysis.
- Feature Extraction: Tokens serve as the basic units for feature extraction in NLP models, allowing algorithms to understand and manipulate text data.
- Normalization: It is important for text normalization as it allows to separate the punctuation, numbers, and special characters from words.
- Vocabulary Management: Tokenization splits the text into words, subwords, and characters which helps to build the vocabulary and word embeddings (numerical representations) capturing the semantic relationship between the tokens.
- Text Preprocessing: Tokenization is often the first step in text preprocessing pipelines, followed by tasks such as stop word removal, stemming or lemmatization, and part-of-speech tagging. It prepares the text data for downstream NLP tasks like sentiment analysis, named entity recognition, and text classification.
- Language Understanding: Tokenization helps algorithms understand the syntactic and semantic structure of the text by representing it as a sequence of tokens. This sequence-based representation is essential for sequence modeling tasks like language modeling, machine translation, and text generation.
Tokenization Techniques
- Word Tokenization: In word tokenization sentences are split into individual words based on whitespace or punctuation.
Example: “Hello, world!” → [“Hello”, “,”, “world”, “!”]
- Sentence Tokenization: In sentence tokenization text is divided into sentences based on punctuation or specific delimiters.
Example: “Hello, world! This is a sentence.” → [“Hello, world!”, “This is a sentence.”]
- Subword Tokenization: Subword tokenization divides words into smaller subword units, useful for handling unknown words or morphologically rich languages.
Example: “unhappy” → [“un”, “happy”]
- Character Tokenization: In character tokenization text is segmented into individual characters, and each character is treated as a separate token.
Example: “hello” → [“h”, “e”, “l”, “l”, “o”]
Implementation of Sentence and Word Tokenization
This section shows the implementation of sentence and word tokenization using the NLTK library.
Word tokenization using NLTK
# Example text for tokenization
text = "Tokenization is important step in NLP."
# Importing NLTK and word tokenizer
import nltk
from nltk.tokenize import word_tokenize
# Word Tokenization
word_tokens = word_tokenize(text)
print("Word tokens:", word_tokens)
Output:
Word tokens: ['Tokenization', 'is', 'important', 'step', 'in', 'NLP', '.']
In the above example, text is split into individual words using the word_tokenizer function from NLTK. It also gave the punction mark (.) as a token and the user can decide to remove it during preprocessing.
Sentence tokenization using NLTK
import nltk
from nltk.tokenize import sent_tokenize
# Example text for tokenization
text = "Tokenization is important step in NLP. It breaks the text into smaller units"
# Sentence Tokenization
sentence_tokens = sent_tokenize(text)
print("Sentence tokens:", sentence_tokens)
Output:
Sentence tokens: ['Tokenization is important step in NLP.', 'It breaks the text into smaller units']
Here sent_tokenize function is used to split the text into individual sentences.